WxPython FAQ Dialogs
Материал из Wiki.crossplatform.ru
Dialog windows or dialogs are an indispensable part of most modern GUI applications. A dialog is defined as a conversation between two or more persons. In a computer application a dialog is a window which is used to "talk" to the application. A dialog is used to input data, modify data, change the application settings etc. Dialogs are important means of communication between a user and a computer program.
Содержание |
A Simple message box
A message box provides short information to the user. A good example is a cd burning application. When a cd is finished burning, a message box pops up.
#!/usr/bin/python # message.py import wx class MessageDialog(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title) wx.FutureCall(5000, self.ShowMessage) self.Centre() self.Show(True) def ShowMessage(self): wx.MessageBox('Download completed', 'Info') app = wx.App() MessageDialog(None, -1, 'MessageDialog') app.MainLoop()
wx.FutureCall(5000, self.ShowMessage)
wx.FutureCall calls a method after 5 seconds. The first parameter is a time value, after which a given method is called. The parameter is in milliseconds. The second parameter is a method to be called.
def ShowMessage(self): wx.MessageBox('Download completed', 'Info')
wx.MessageBox shows a small dialog window. We provide three parameters. The text message, the title message and finally the button.
Predefined dialogs
wxPython has several predefined dialogs. These are dialogs for common programming tasks like showing text, receiving input , loading and saving files etc.
Message dialogs
Message dialogs are used to show messages to the user. They are more flexible than simple message boxes, that we saw in the previous example. They are customizable. We can change icons and buttons that will be shown in a dialog.
wx.MessageDialog(wx.Window parent, string message, string caption=wx.MessageBoxCaptionStr, long style=wx.OK | wx.CANCEL | wx.CENTRE, wx.Point pos=(-1, -1))
flag | meaning |
---|---|
wx.OK | show Ok button |
wx.CANCEL | show Cancel button |
wx.YES_NO | show Yes, No buttons |
wx.YES_DEFAULT | make Yes button the default |
wx.NO_DEFAULT | make No button the default |
wx.ICON_EXCLAMATION | show an alert icon |
wx.ICON_ERROR | show an error icon |
wx.ICON_HAND | same as wx.ICON_ERROR |
wx.ICON_INFORMATION | show an info icon |
wx.ICON_QUESTION | show a question icon |
#!/usr/bin/python # messages.py import wx class Messages(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, size=(250, 150)) panel = wx.Panel(self, -1) hbox = wx.BoxSizer() sizer = wx.GridSizer(2, 2, 2, 2) btn1 = wx.Button(panel, -1, 'Info') btn2 = wx.Button(panel, -1, 'Error') btn3 = wx.Button(panel, -1, 'Question') btn4 = wx.Button(panel, -1, 'Alert') sizer.AddMany([btn1, btn2, btn3, btn4]) hbox.Add(sizer, 0, wx.ALL, 15) panel.SetSizer(hbox) btn1.Bind(wx.EVT_BUTTON, self.ShowMessage1) btn2.Bind(wx.EVT_BUTTON, self.ShowMessage2) btn3.Bind(wx.EVT_BUTTON, self.ShowMessage3) btn4.Bind(wx.EVT_BUTTON, self.ShowMessage4) self.Centre() self.Show(True) def ShowMessage1(self, event): dial = wx.MessageDialog(None, 'Download completed', 'Info', wx.OK) dial.ShowModal() def ShowMessage2(self, event): dial = wx.MessageDialog(None, 'Error loading file', 'Error', wx.OK | wx.ICON_ERROR) dial.ShowModal() def ShowMessage3(self, event): dial = wx.MessageDialog(None, 'Are you sure to quit?', 'Question', wx.YES_NO | wx.NO_DEFAULT | wx.ICON_QUESTION) dial.ShowModal() def ShowMessage4(self, event): dial = wx.MessageDialog(None, 'Unallowed operation', 'Exclamation', wx.OK | wx.ICON_EXCLAMATION) dial.ShowModal() app = wx.App() Messages(None, -1, 'Messages') app.MainLoop()
In our example, we have created four buttons and put them in a grid sizer. These buttons will show four different dialog windows. We create them by specifying different style flags.
dial = wx.MessageDialog(None, 'Error loading file', 'Error', wx.OK | wx.ICON_ERROR) dial.ShowModal()
The creation of the message dialog is simple. We set the dialog to be a toplevel window by providing None as a parent. The two strings provide the message text and the dialog title. We show an ok button and an error icon by specifying the wx.OK and wx.ICON_ERROR flags. To show the dialog on screen, we call the ShowModal() method.
About dialog box
Almost every application has a typical about dialog box. It is usually placed in the Help menu. The purpose of this dialog is to give the user the basic information about the name and the version of the application. In the past, these dialogs used to be quite brief. These days most of these boxes provide additional information about the authors. They give credits to additional programmers or documentation writers. They also provide information about the application licence. These boxes can show the logo of the company or the application logo. Some of the more capable about boxes show animation. wxPython has a special about dialog box starting from 2.8.x series. It is not rocket science to make such a dialog manually. But it makes a programmer's life easier.
The dialog box is located in the Misc module. In order to create an about dialog box we must create two objects. A wx.AboutDialogInfo and a wx.AboutBox.
wx.AboutDialogInfo()
We will call the following methods upon a wx.AboutDialogInfo object in our example. These methods are self-exlanatory.
Method | Description |
---|---|
SetName(string name) | set the name of the program |
SetVersion(string version) | set the version of the program |
SetDescription(string desc) | set the description of the program |
SetCopyright(string copyright) | set the copyright fo the program |
SetLicence(string licence) | set the licence of the program |
SetIcon(wx.Icon icon) | set the icon to be show |
SetWebSite(string URL) | set the website of the program |
SetLicence(string licence) | set the licence of the program |
AddDeveloper(string developer) | add a developer to the developer's list |
AddDocWriter(string docwirter) | add a docwriter to the docwriter's list |
AddArtist(string artist) | add an artist to the artist's list |
AddTranslator(string developer) | add a developer to the translator's list |
The constructor of the wx.AboutBox is as follows. It takes a wx.AboutDialogInfo as a parameter.
wx.AboutBox(wx.AboutDialogInfo info)
wxPython can display two kinds of About boxes. It depends on which platform we use and which methods we call.
It can be a native dialog or a wxPython generic dialog. Windows native about dialog box cannot display custom icons, licence text nor the url's. If we omit these three fields, wx.Python will show a native dialog. Otherwise it will resort to a generic one. It is advised to provide licence information in a separate menu item, if we want to stay as native as possible.
GTK+ can show all these fields.
#!/usr/bin/python # aboutbox.py import wx ID_ABOUT = 1 class AboutDialogBox(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, size=(260, 200)) menubar = wx.MenuBar() help = wx.Menu() help.Append(ID_ABOUT, '&About') self.Bind(wx.EVT_MENU, self.OnAboutBox, id=ID_ABOUT) menubar.Append(help, '&Help') self.SetMenuBar(menubar) self.Centre() self.Show(True) def OnAboutBox(self, event): description = """File Hunter is an advanced file manager for the Unix operating system. Features include powerful built-in editor, advanced search capabilities, powerful batch renaming, file comparison, extensive archive handling and more. """ licence = """File Hunter is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version. File Hunter is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. You should have received a copy of the GNU General Public License along with File Hunter; if not, write to the Free Software Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA""" info = wx.AboutDialogInfo() info.SetIcon(wx.Icon('icons/hunter.png', wx.BITMAP_TYPE_PNG)) info.SetName('File Hunter') info.SetVersion('1.0') info.SetDescription(description) info.SetCopyright('(C) 2007 jan bodnar') info.SetWebSite('http://www.zetcode.com') info.SetLicence(licence) info.AddDeveloper('jan bodnar') info.AddDocWriter('jan bodnar') info.AddArtist('The Tango crew') info.AddTranslator('jan bodnar') wx.AboutBox(info) app = wx.App() AboutDialogBox(None, -1, 'About dialog box') app.MainLoop()
description = """File Hunter is an advanced file manager for the Unix operating
system. Features include powerful built-in editor, advanced search capabilities,
powerful batch renaming, file comparison, extensive archive handling and more.
"""
It is not the best idea to put too much text into the code of the application. I don't want to make the example too complex, so I put all the text into the code. But in real world programs, the text should be placed separately inside a file. It helps us with the maintenace of our application. For example, if we want to translate our application to other languages.
info = wx.AboutDialogInfo()
The first thing to do is to create a wx.AboutDialogInfo object. The constructor is empty. It does not taky any parameters.
info.SetIcon(wx.Icon('icons/hunter.png', wx.BITMAP_TYPE_PNG)) info.SetName('File Hunter') info.SetVersion('1.0') info.SetDescription(description) info.SetCopyright('(C) 2007 jan bodnar') info.SetWebSite('http://www.zetcode.com') info.SetLicence(licence) info.AddDeveloper('jan bodnar') info.AddDocWriter('jan bodnar') info.AddArtist('The Tango crew') info.AddTranslator('jan bodnar')
The next thing to do is to call all necessary methods upon the created wx.AboutDialogInfo object.
wx.AboutBox(info)
In the end we create a wx.AboutBox widget. The only parameter it takes is the wx.AboutDialogInfo object.
And of course, if we want to have an animation or some other eye candy, we must implement our about dialog manually.
A custom dialog
In the next example we create a custom dialog. An image editing application can change a color depth of a picture. To provide this funcionality, we could create a suitable dialog.
#!/usr/bin/python # colordepth.py import wx ID_DEPTH = 1 class ChangeDepth(wx.Dialog): def __init__(self, parent, id, title): wx.Dialog.__init__(self, parent, id, title, size=(250, 210)) panel = wx.Panel(self, -1) vbox = wx.BoxSizer(wx.VERTICAL) wx.StaticBox(panel, -1, 'Colors', (5, 5), (240, 150)) wx.RadioButton(panel, -1, '256 Colors', (15, 30), style=wx.RB_GROUP) wx.RadioButton(panel, -1, '16 Colors', (15, 55)) wx.RadioButton(panel, -1, '2 Colors', (15, 80)) wx.RadioButton(panel, -1, 'Custom', (15, 105)) wx.TextCtrl(panel, -1, '', (95, 105)) hbox = wx.BoxSizer(wx.HORIZONTAL) okButton = wx.Button(self, -1, 'Ok', size=(70, 30)) closeButton = wx.Button(self, -1, 'Close', size=(70, 30)) hbox.Add(okButton, 1) hbox.Add(closeButton, 1, wx.LEFT, 5) vbox.Add(panel) vbox.Add(hbox, 1, wx.ALIGN_CENTER | wx.TOP | wx.BOTTOM, 10) self.SetSizer(vbox) class ColorDepth(wx.Frame): def __init__(self, parent, id, title): wx.Frame.__init__(self, parent, id, title, size=(350, 220)) toolbar = self.CreateToolBar() toolbar.AddLabelTool(ID_DEPTH, '', wx.Bitmap('icons/color.png')) self.Bind(wx.EVT_TOOL, self.OnChangeDepth, id=ID_DEPTH) self.Centre() self.Show(True) def OnChangeDepth(self, event): chgdep = ChangeDepth(None, -1, 'Change Color Depth') chgdep.ShowModal() chgdep.Destroy() app = wx.App() ColorDepth(None, -1, '') app.MainLoop()
class ChangeDepth(wx.Dialog): def __init__(self, parent, id, title): wx.Dialog.__init__(self, parent, id, title, size=(250, 210))
In our code example we create a custom ChangeDepth dialog. We inherit from a wx.Dialog widget.
chgdep = ChangeDepth(None, -1, 'Change Color Depth') chgdep.ShowModal() chgdep.Destroy()
We instantiate a ChangeDepth class. Then we call the ShowModal() dialog. We must not forget to destroy our dialog. Notice the visual difference between the dialog and the top level window. The dialog in the following figure has been activated. We cannot work with the toplevel window until the dialog is destroyed. There is a clear difference in the titlebar of the windows.
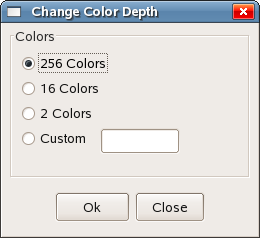
